Automated email alerts can be incredibly useful for monitoring data and notifying stakeholders of important changes or thresholds. Here’s a guide on how to set up automated email alerts using Google Sheets and Excel.
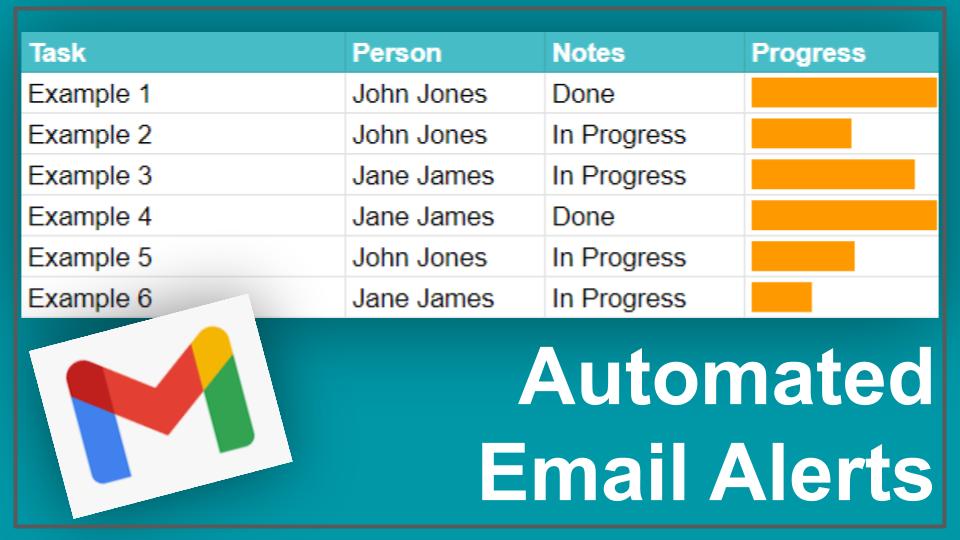
Automated email alerts can be incredibly useful for monitoring data and notifying stakeholders of important changes or thresholds. Here’s a guide on how to set up automated email alerts using Google Sheets and Excel.
Google Sheets: Google Apps Script
Google Apps Script allows you to create automated email alerts based on conditions in your Google Sheets. Below is a basic example of how you can set this up.
Example: Send Email Alerts Based on Thresholds
Script:
function sendEmailAlerts() {
var sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet();
var range = sheet.getRange('B1:B10'); // Range to check
var values = range.getValues();
for (var i = 0; i < values.length; i++) {
if (values[i][0] > 100) { // Condition for sending an alert
MailApp.sendEmail({
to: '[email protected]',
subject: 'Alert: Value Exceeds Threshold',
body: 'The value in cell B' + (i + 1) + ' exceeds the threshold. Current value: ' + values[i][0]
});
}
}
}
Steps to Set Up:
- Open Google Sheets and go to
Extensions
>Apps Script
. - Paste the Script into the code editor.
- Save the script and give it a name.
- To run the script automatically, go to
Triggers
(clock icon on the left), click+ Add Trigger
, choosesendEmailAlerts
as the function to run, and set up the desired frequency (e.g., daily, hourly).
Excel: VBA and Office Scripts
VBA (Visual Basic for Applications)
In Excel, VBA allows you to set up email alerts that can be triggered by specific conditions. Here’s a VBA example for sending automated email alerts.
Example: Send Email Alerts Based on Thresholds
VBA Script:
Sub SendEmailAlerts()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Sheet1")
Dim rng As Range
Set rng = ws.Range("B1:B10") ' Range to check
Dim cell As Range
For Each cell In rng
If cell.Value > 100 Then ' Condition for sending an alert
Dim OutlookApp As Object
Dim OutlookMail As Object
Set OutlookApp = CreateObject("Outlook.Application")
Set OutlookMail = OutlookApp.CreateItem(0)
With OutlookMail
.To = "[email protected]"
.Subject = "Alert: Value Exceeds Threshold"
.Body = "The value in cell B" & cell.Row & " exceeds the threshold. Current value: " & cell.Value
.Send
End With
Set OutlookMail = Nothing
Set OutlookApp = Nothing
End If
Next cell
End Sub
Steps to Set Up:
- Open Excel and press
ALT + F11
to open the VBA editor. - Insert a New Module by right-clicking on any existing module or the project tree, then selecting
Insert
>Module
. - Paste the VBA Script into the module.
- Save the VBA project.
- To automate, you can set up a scheduled task in Windows Task Scheduler or use a workbook event like
Workbook_Open()
to run the script.
Office Scripts (Excel for the Web)
Office Scripts is a modern scripting option for Excel on the web, using TypeScript or JavaScript. Here’s how to send email alerts using Office Scripts.
Example: Send Email Alerts Based on Thresholds
Office Script:
async function main(workbook: ExcelScript.Workbook) {
let sheet = workbook.getActiveWorksheet();
let range = sheet.getRange("B1:B10"); // Range to check
let values = range.getValues();
for (let i = 0; i < values.length; i++) {
if (values[i][0] > 100) { // Condition for sending an alert
await Office.context.mailbox.item.notificationMessages.addAsync(
{
type: "informational",
message: `The value in cell B${i + 1} exceeds the threshold. Current value: ${values[i][0]}`
}
);
}
}
}
Steps to Set Up:
- Open Excel Online and go to
Automate
>All Scripts
. - Create a New Script and paste the code into the script editor.
- Save the script with a name.
- To automate, set up a trigger using Power Automate to run this script based on your desired schedule.
Automating email alerts can significantly enhance your ability to monitor and respond to changes in your spreadsheet data. Whether you use Google Apps Script for Google Sheets or VBA/Office Scripts for Excel, these examples provide a solid foundation to build upon and customize according to your specific needs.